Whilst I have put React utility, there is not the sort of factor as React utility. I imply, there are
front-end packages written in JavaScript or TypeScript that occur to
use React as their perspectives. On the other hand, I believe it isn’t honest to name them React
packages, simply as we would not name a Java EE utility JSP
utility.
Extra incessantly than no longer, other people squeeze various things into React
parts or hooks to make the appliance paintings. This kind of
less-organised construction is not an issue if the appliance is small or
most commonly with out a lot trade common sense. On the other hand, as extra trade common sense shifted
to front-end in lots of circumstances, this everything-in-component presentations issues. To
be extra explicit, the trouble of working out such form of code is
rather excessive, in addition to the larger chance to code amendment.
On this article, I want to speak about a couple of patterns and methods
you’ll use to reshape your âReact utilityâ into a normal one, and simplest
with React as its view (you’ll even change those perspectives into any other view
library with out an excessive amount of efforts).
The crucial level this is you must analyse what position every a part of the
code is enjoying inside an utility (even at the floor, they may well be
packed in the similar document). Separate view from no-view common sense, break up the
no-view common sense additional by way of their tasks and position them within the
proper puts.
The good thing about this separation is that it lets you make adjustments in
the underlying area common sense with out being worried an excessive amount of in regards to the floor
perspectives, or vice versa. Additionally, it could build up the reusability of the area
common sense elsewhere as they aren’t coupled to every other portions.
React is a humble library for construction perspectives
It is simple to put out of your mind that React, at its core, is a library (no longer a
framework) that is helping you construct the person interface.
On this context, it’s emphasised that React is a JavaScript library
that concentrates on a selected facet of internet construction, particularly UI
parts, and provides plentiful freedom with regards to the design of the
utility and its general construction.
A JavaScript library for construction person interfaces
It should sound lovely easy. However I’ve noticed many circumstances the place
other people write the knowledge fetching, reshaping common sense proper within the position the place
it is ate up. As an example, fetching records inside of a React factor, within the
useEffect
block proper above the rendering, or acting records
mapping/remodeling after they were given the reaction from the server aspect.
useEffect(() => { fetch("https://deal with.provider/api") .then((res) => res.json()) .then((records) => { const addresses = records.map((merchandise) => ({ side road: merchandise.streetName, deal with: merchandise.streetAddress, postcode: merchandise.postCode, })); setAddresses(addresses); }); }, []); // the real rendering...
Most likely as a result of there’s but to be a common usual within the frontend
global, or it is only a dangerous programming dependancy. Frontend packages must
no longer be handled too otherwise from common tool packages. Within the
frontend global, you continue to use separation of considerations typically to organize
the code construction. And all of the shown helpful design patterns nonetheless
follow.
Welcome to the actual global React utility
Maximum builders had been inspired by way of React’s simplicity and the concept
a person interface can also be expressed as a natural serve as to map records into the
DOM. And to a definite extent, it IS.
However builders begin to battle after they want to ship a community
request to a backend or carry out web page navigation, as those unwanted effects
make the factor much less ânaturalâ. And whenever you believe those other
states (both international state or native state), issues briefly get
difficult, and the darkish aspect of the person interface emerges.
With the exception of the person interface
React itself doesnât care a lot about the place to position calculation or
trade common sense, which is honest because itâs just a library for construction person
interfaces. And past that view layer, a frontend utility has different
portions as nicely. To make the appliance paintings, you’re going to want a router,
native garage, cache at other ranges, community requests, Third-party
integrations, Third-party login, safety, logging, efficiency tuning,
and many others.
With all this additional context, looking to squeeze every thing into
React parts or hooks is normally no longer a good suggestion. The reason being
blending ideas in a single position normally ends up in extra confusion. At
first, the factor units up some community request for order standing, and
then there’s some common sense to trim off main house from a string and
then navigate in different places. The reader should continuously reset their
common sense drift and soar backward and forward from other ranges of main points.
Packing all of the code into parts might paintings in small packages
like a Todo or one-form utility. Nonetheless, the efforts to grasp
such utility will probably be important as soon as it reaches a definite point.
To not point out including new options or solving present defects.
If lets separate other considerations into recordsdata or folders with
constructions, the psychological load required to grasp the appliance would
be considerably diminished. And also you simplest have to concentrate on something at a
time. Fortuitously, there are already some well-proven patterns again to the
pre-web time. Those design ideas and patterns are explored and
mentioned nicely to unravel the typical person interface issues – however within the
desktop GUI utility context.
Martin Fowler has a really perfect abstract of the idea that of view-model-data
layering.
At the entire I have discovered this to be an efficient type of
modularization for plenty of packages and person who I incessantly use and
inspire. It is largest merit is that it permits me to extend my
focal point by way of permitting me to take into consideration the 3 subjects (i.e., view,
mannequin, records) rather independently.
Layered architectures had been used to manage the demanding situations in huge
GUI packages, and unquestionably we will use those established patterns of
front-end group in our âReact packagesâ.
The evolution of a React utility
For small or one-off tasks, it’s possible you’ll to find that every one common sense is simply
written inside of React parts. You may even see one or just a few parts
in general. The code appears lovely just like HTML, with only a few variable or
state used to make the web page âdynamicâ. Some may ship requests to fetch
records on useEffect
after the parts render.
As the appliance grows, and an increasing number of code are added to codebase.
With no right kind approach to organise them, quickly the codebase will become
unmaintainable state, which means that even including small options can also be
time-consuming as builders want extra time to learn the code.
So Iâll record a couple of steps that may lend a hand to reduction the maintainable
downside. It normally require just a little extra efforts, however it’s going to repay to
have the construction in you utility. Letâs have a handy guide a rough evaluate of those
steps to construct front-end packages that scale.
Unmarried Element Software
It may be known as just about a Unmarried Element Software:
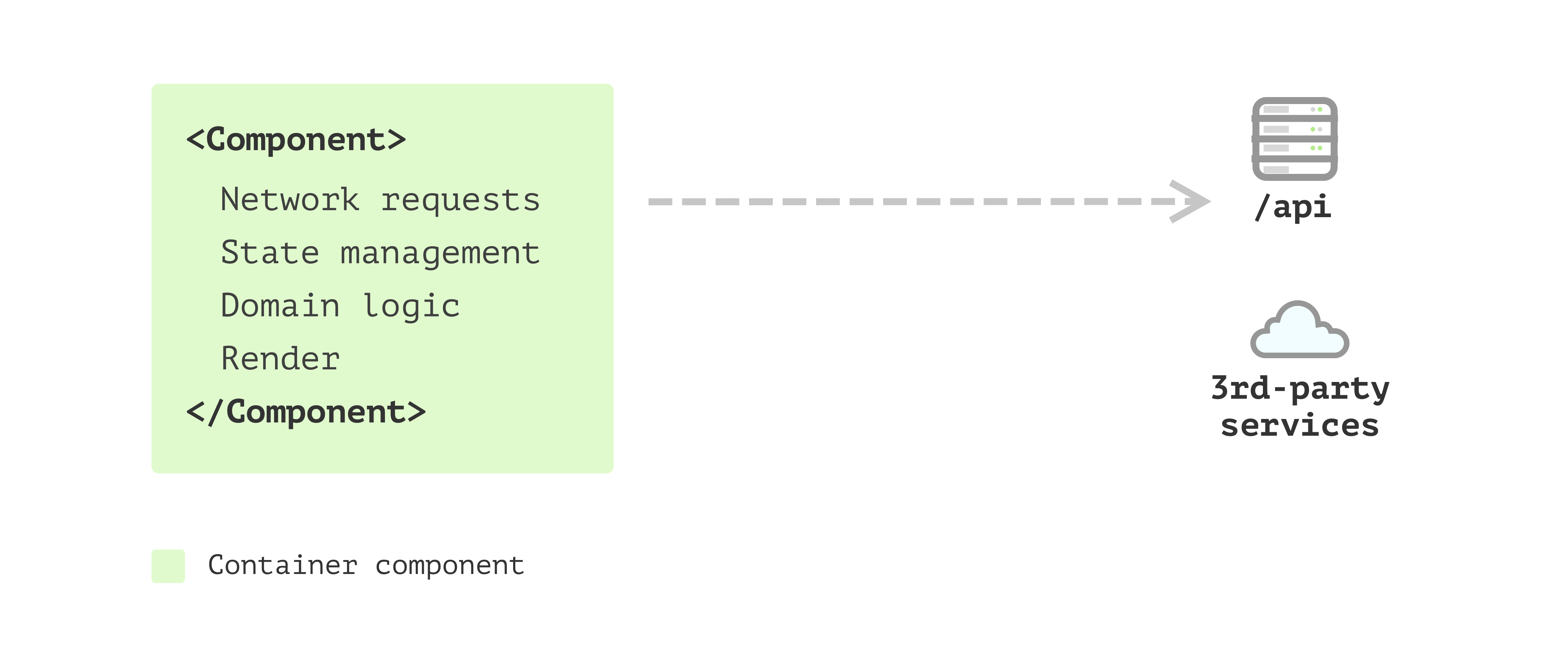
Determine 1: Unmarried Element Software
However quickly, you realise one unmarried factor calls for a large number of time
simply to learn what’s going on. As an example, there’s common sense to iterate
thru a listing and generate every merchandise. Additionally, there’s some common sense for
the usage of Third-party parts with just a few configuration code, aside
from different common sense.
More than one Element Software
You made a decision to separate the factor into a number of parts, with
those constructions reflecting whatâs going down at the outcome HTML is a
just right concept, and it is helping you to concentrate on one factor at a time.
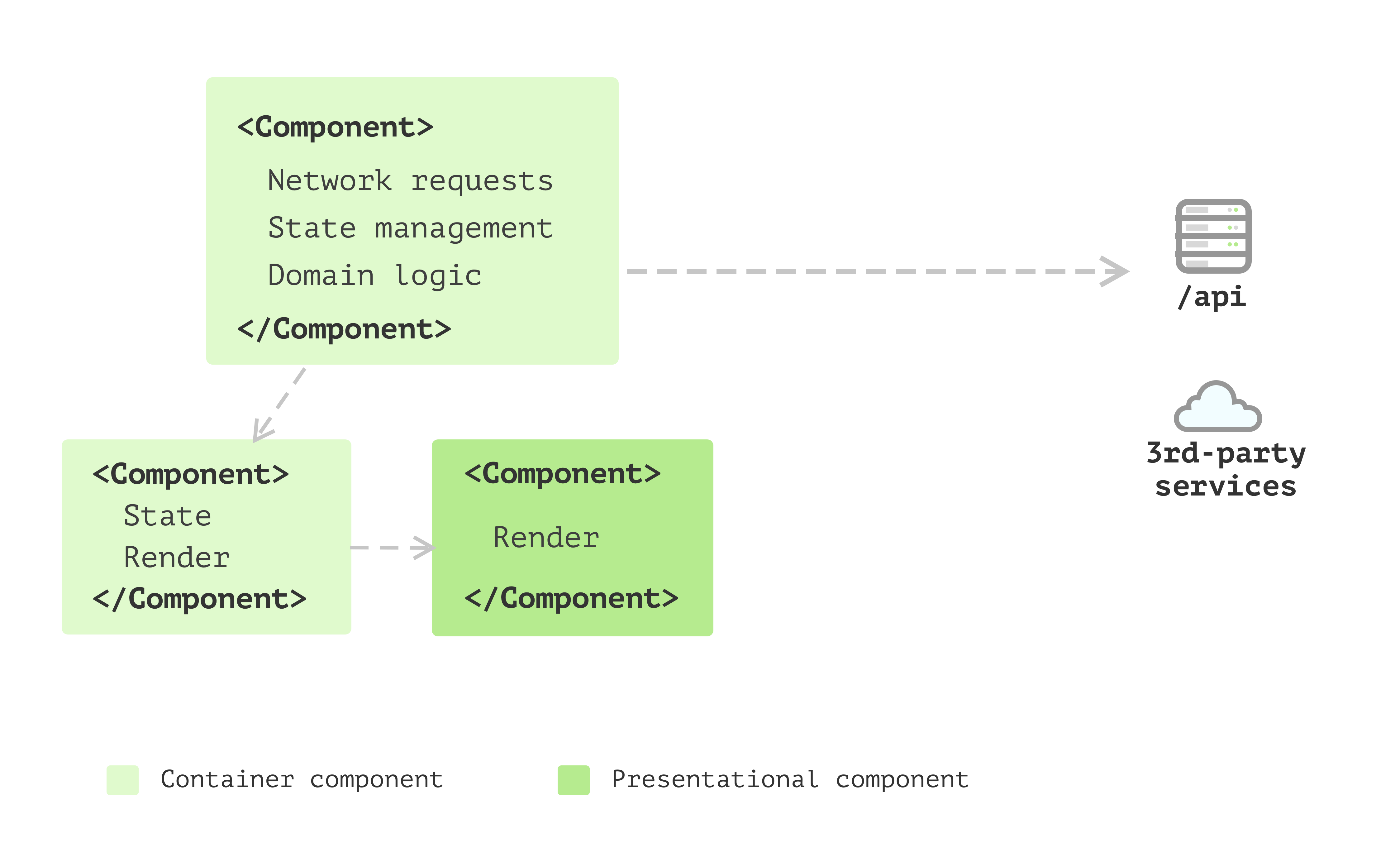
Determine 2: More than one Element Software
And as your utility grows, with the exception of the view, there are issues
like sending community requests, changing records into other shapes for
the view to devour, and amassing records to ship again to the server. And
having this code inside of parts doesnât really feel proper as theyâre no longer
truly about person interfaces. Additionally, some parts have too many
interior states.
State control with hooks
Itâs a greater concept to separate this common sense right into a separate puts.
Fortuitously in React, you’ll outline your individual hooks. It is a nice approach to
percentage those state and the common sense of each time states trade.
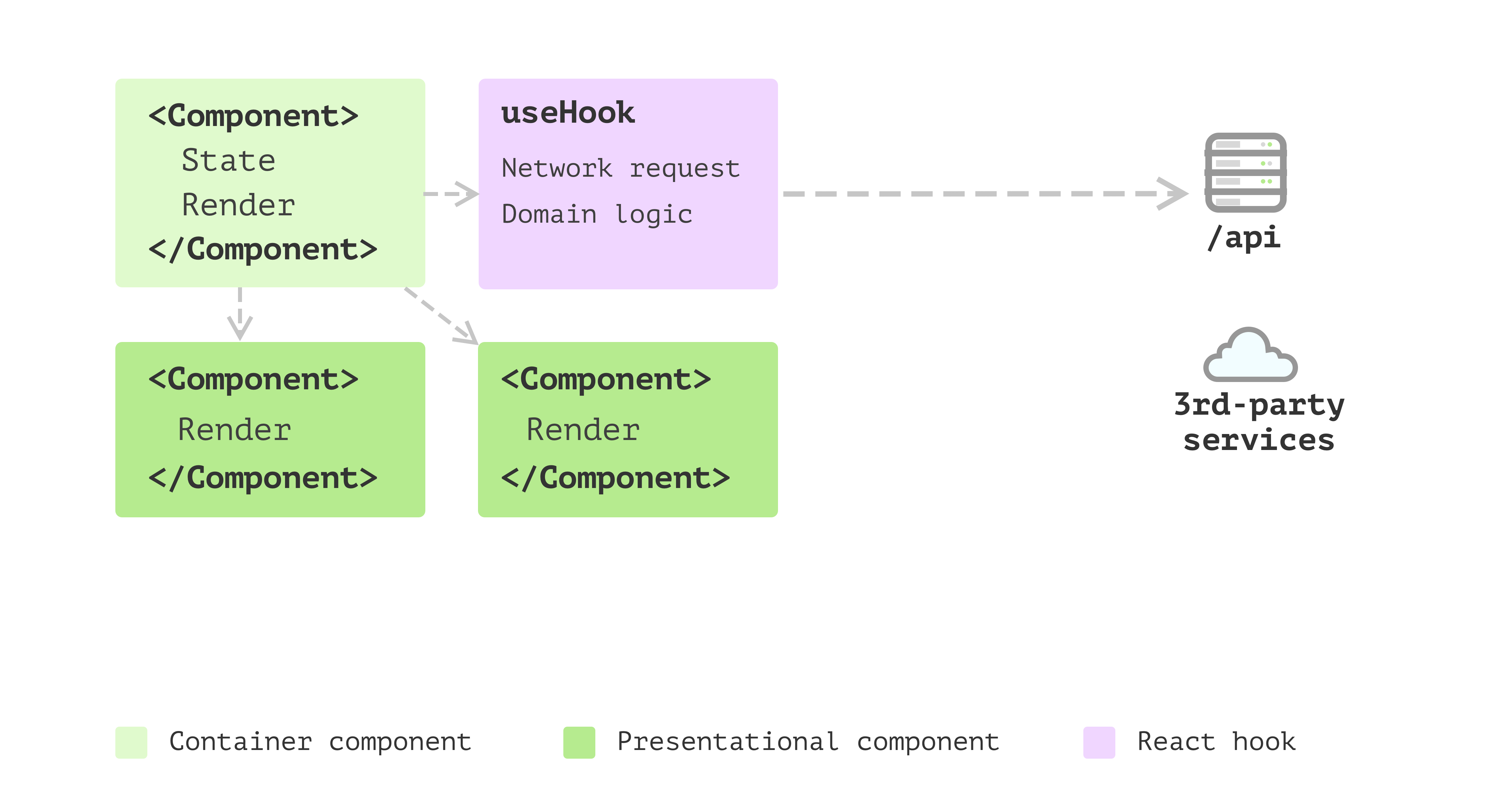
Determine 3: State control with hooks
Thatâs superior! You will have a number of parts extracted out of your
unmarried factor utility, and you have got a couple of natural presentational
parts and a few reusable hooks that make different parts stateful.
The one downside is that during hooks, with the exception of the aspect impact and state
control, some common sense doesnât appear to belong to the state control
however natural calculations.
Trade fashions emerged
So that youâve began to transform conscious that extracting this common sense into but
any other position can deliver you several advantages. As an example, with that break up,
the common sense can also be cohesive and unbiased of any perspectives. Then you definitely extract
a couple of area gadgets.
Those easy gadgets can care for records mapping (from one layout to
any other), test nulls and use fallback values as required. Additionally, because the
quantity of those area gadgets grows, you to find you want some inheritance
or polymorphism to make issues even cleaner. Thus you implemented many
design patterns you discovered useful from different puts into the front-end
utility right here.
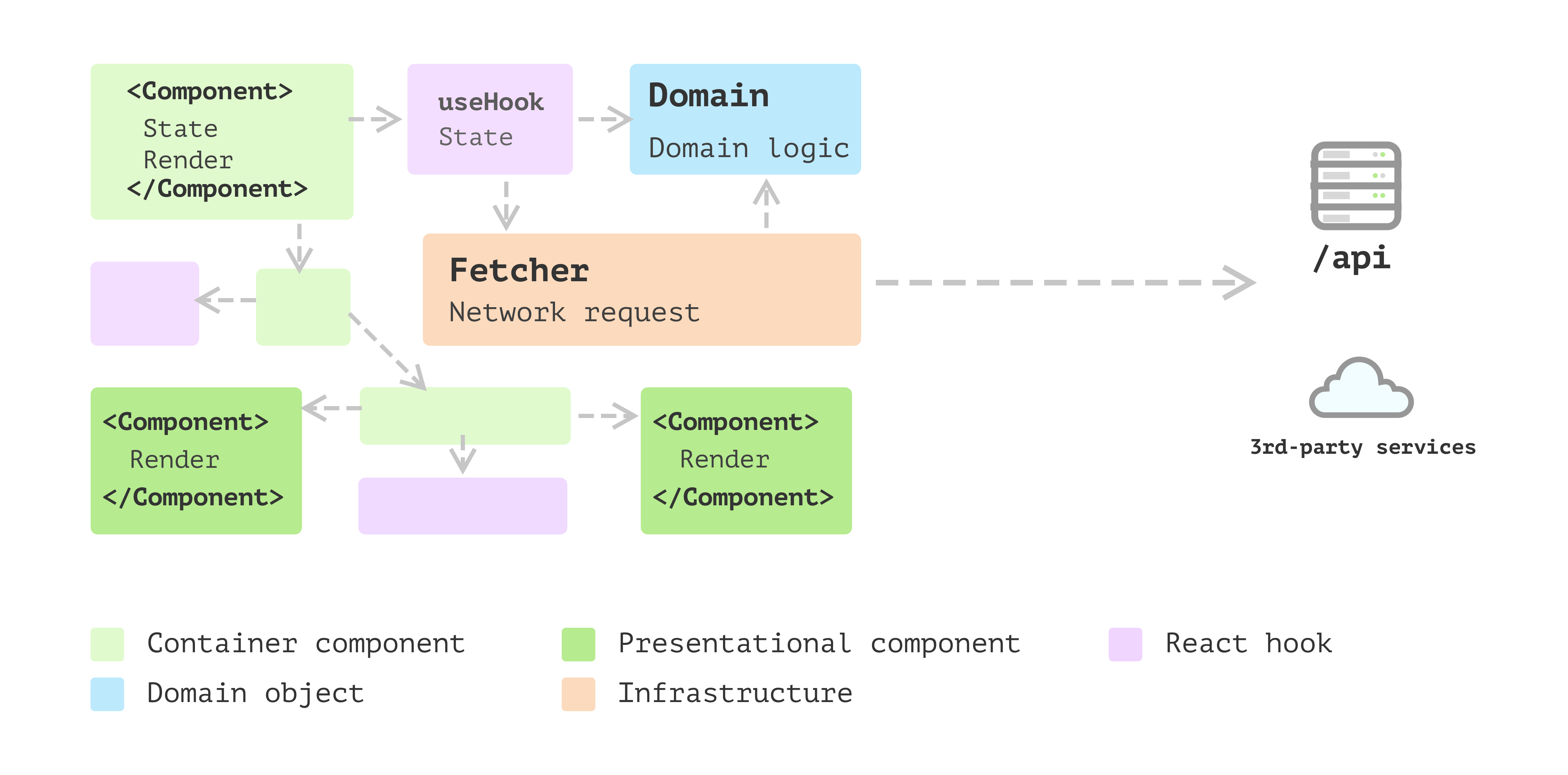
Determine 4: Trade fashions
Layered frontend utility
The applying helps to keep evolving, and then you definately to find some patterns
emerge. There are a number of gadgets that don’t belong to any person
interface, and so they additionally donât care about whether or not the underlying records is
from far off provider, native garage or cache. After which, you wish to have to separate
them into other layers. Here’s a detailed rationalization in regards to the layer
splitting Presentation Area Knowledge Layering.
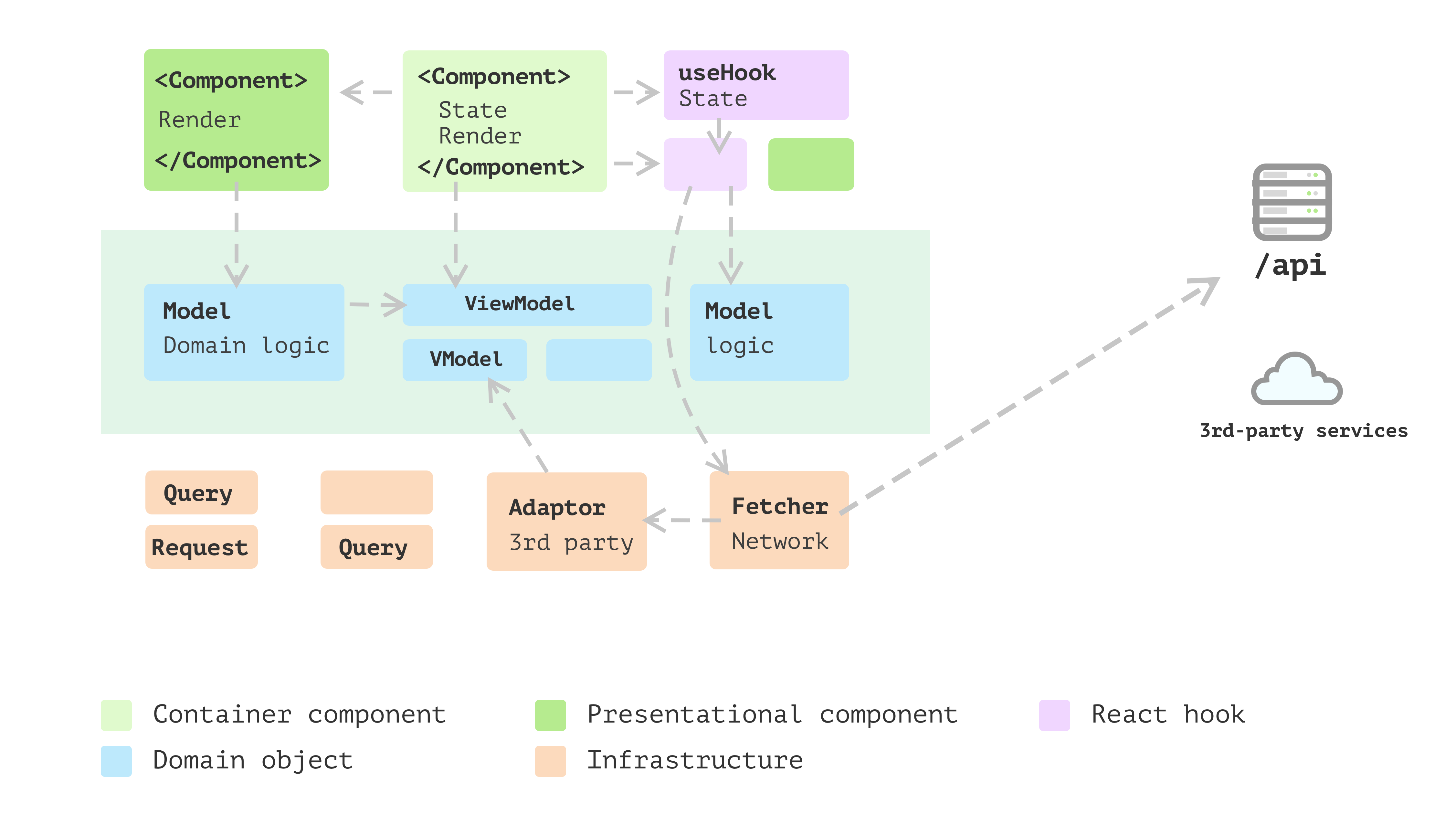
Determine 5: Layered frontend utility
The above evolution procedure is a high-level evaluate, and also you must
have a style of ways you must construction your code or a minimum of what the
path must be. On the other hand, there will probably be many main points you want to
believe ahead of making use of the speculation to your utility.
Within the following sections, Iâll stroll you thru a function I
extracted from an actual undertaking to show all of the patterns and design
ideas I believe helpful for giant frontend packages.
Creation of the Fee function
Iâm the usage of an oversimplified on-line ordering utility as a beginning
level. On this utility, a buyer can select up some merchandise and upload
them to the order, after which they are going to want to choose one of the vital fee
how you can proceed.
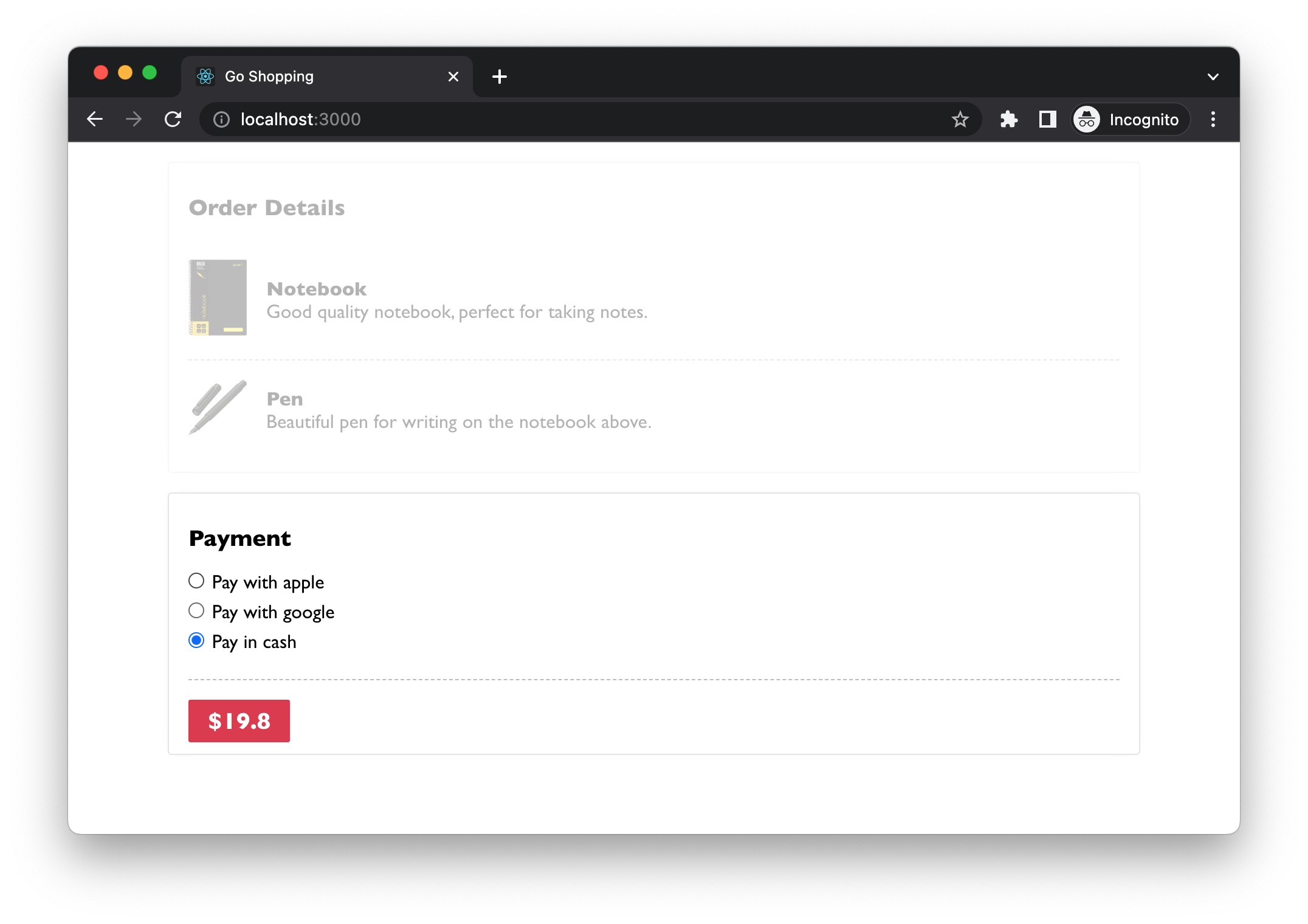
Determine 6: Fee phase
Those fee approach choices are configured at the server aspect, and
consumers from other nations might see different choices. As an example,
Apple Pay might simplest be in style in some nations. The radio buttons are
data-driven – no matter is fetched from the backend provider will probably be
surfaced. The one exception is that after no configured fee tricks
are returned, we donât display anything else and deal with it as âpay in money” by way of
default.
For simplicity, Iâll skip the real fee procedure and concentrate on the
Fee
factor. Letâs say that once studying the React hi global
document and a few stackoverflow searches, you got here up with some code
like this:
src/Fee.tsxâ¦
export const Fee = ({ quantity }: { quantity: quantity }) => { const [paymentMethods, setPaymentMethods] = useState<LocalPaymentMethod[]>( [] ); useEffect(() => { const fetchPaymentMethods = async () => { const url = "https://online-ordering.com/api/payment-methods"; const reaction = look forward to fetch(url); const tricks: RemotePaymentMethod[] = look forward to reaction.json(); if (tricks.period > 0) { const prolonged: LocalPaymentMethod[] = tricks.map((approach) => ({ supplier: approach.title, label: `Pay with ${approach.title}`, })); prolonged.push({ supplier: "money", label: "Pay in money" }); setPaymentMethods(prolonged); } else { setPaymentMethods([]); } }; fetchPaymentMethods(); }, []); go back ( <div> <h3>Fee</h3> <div> {paymentMethods.map((approach) => ( <label key={approach.supplier}> <enter kind="radio" title="fee" worth={approach.supplier} defaultChecked={approach.supplier === "money"} /> <span>{approach.label}</span> </label> ))} </div> <button>${quantity}</button> </div> ); };
The code above is lovely standard. You’ll have noticed it within the get
began educational someplace. And it isn’t essential dangerous. On the other hand, as we
discussed above, the code has blended other considerations all in one
factor and makes it just a little tricky to learn.
The issue with the preliminary implementation
The primary factor I want to deal with is how busy the factor
is. By way of that, I imply Fee
offers with various things and makes the
code tricky to learn as it’s important to transfer context to your head as you
learn.
With the intention to make any adjustments it’s important to comprehend
learn how to initialise community request
,
learn how to map the knowledge to a neighborhood layout that the factor can perceive
,
learn how to render every fee approach
,
and
the rendering common sense for Fee
factor itself
.
src/Fee.tsxâ¦
export const Fee = ({ quantity }: { quantity: quantity }) => { const [paymentMethods, setPaymentMethods] = useState<LocalPaymentMethod[]>( [] ); useEffect(() => { const fetchPaymentMethods = async () => { const url = "https://online-ordering.com/api/payment-methods"; const reaction = look forward to fetch(url); const tricks: RemotePaymentMethod[] = look forward to reaction.json(); if (tricks.period > 0) { const prolonged: LocalPaymentMethod[] = tricks.map((approach) => ({ supplier: approach.title, label: `Pay with ${approach.title}`, })); prolonged.push({ supplier: "money", label: "Pay in money" }); setPaymentMethods(prolonged); } else { setPaymentMethods([]); } }; fetchPaymentMethods(); }, []); go back ( <div> <h3>Fee</h3> <div> {paymentMethods.map((approach) => ( <label key={approach.supplier}> <enter kind="radio" title="fee" worth={approach.supplier} defaultChecked={approach.supplier === "money"} /> <span>{approach.label}</span> </label> ))} </div> <button>${quantity}</button> </div> ); };
It isn’t a large downside at this degree for this easy instance.
On the other hand, because the code will get larger and extra advanced, we will want to
refactoring them just a little.
Itâs just right follow to separate view and non-view code into separate
puts. The reason being, typically, perspectives are converting extra often than
non-view common sense. Additionally, as they handle other sides of the
utility, setting apart them lets you focal point on a selected
self-contained module this is a lot more manageable when enforcing new
options.
The break up of view and non-view code
In React, we will use a customized hook to take care of state of an element
whilst conserving the factor itself roughly stateless. We will be able to
use
to create a serve as known as usePaymentMethods
(the
prefix use
is a tradition in React to signify the serve as is a hook
and dealing with some states in it):
src/Fee.tsxâ¦
const usePaymentMethods = () => {
const [paymentMethods, setPaymentMethods] = useState<LocalPaymentMethod[]>(
[]
);
useEffect(() => {
const fetchPaymentMethods = async () => {
const url = "https://online-ordering.com/api/payment-methods";
const reaction = look forward to fetch(url);
const tricks: RemotePaymentMethod[] = look forward to reaction.json();
if (tricks.period > 0) {
const prolonged: LocalPaymentMethod[] = tricks.map((approach) => ({
supplier: approach.title,
label: `Pay with ${approach.title}`,
}));
prolonged.push({ supplier: "money", label: "Pay in money" });
setPaymentMethods(prolonged);
} else {
setPaymentMethods([]);
}
};
fetchPaymentMethods();
}, []);
go back {
paymentMethods,
};
};
This returns a paymentMethods
array (in kind LocalPaymentMethod
) as
interior state and is in a position for use in rendering. So the common sense in
Fee
can also be simplified as:
src/Fee.tsxâ¦
export const Fee = ({ quantity }: { quantity: quantity }) => {
const { paymentMethods } = usePaymentMethods();
go back (
<div>
<h3>Fee</h3>
<div>
{paymentMethods.map((approach) => (
<label key={approach.supplier}>
<enter
kind="radio"
title="fee"
worth={approach.supplier}
defaultChecked={approach.supplier === "money"}
/>
<span>{approach.label}</span>
</label>
))}
</div>
<button>${quantity}</button>
</div>
);
};
This is helping relieve the ache within the Fee
factor. On the other hand, should you
take a look at the block for iterating thru paymentMethods
, it sort of feels a
idea is lacking right here. In different phrases, this block merits its personal
factor. Preferably, we wish every factor to concentrate on, just one
factor.
Knowledge modelling to encapsulate common sense
Thus far, the adjustments we have now made are all about splitting view and
non-view code into other puts. It really works nicely. The hook handles records
fetching and reshaping. Each Fee
and PaymentMethods
are rather
small and simple to grasp.
On the other hand, should you appearance carefully, there’s nonetheless room for development. To
get started with, within the natural serve as factor PaymentMethods
, we have now just a little
of common sense to test if a fee approach must be checked by way of default:
src/Fee.tsxâ¦
const PaymentMethods = ({
paymentMethods,
}: {
paymentMethods: LocalPaymentMethod[];
}) => (
<>
{paymentMethods.map((approach) => (
<label key={approach.supplier}>
<enter
kind="radio"
title="fee"
worth={approach.supplier}
defaultChecked={approach.supplier === "money"}
/>
<span>{approach.label}</span>
</label>
))}
</>
);
Those take a look at statements in a view can also be regarded as a common sense leak, and
steadily they may be able to be scatted elsewhere and make amendment
tougher.
Some other level of doable common sense leakage is within the records conversion
the place we fetch records:
src/Fee.tsxâ¦
const usePaymentMethods = () => { const [paymentMethods, setPaymentMethods] = useState<LocalPaymentMethod[]>( [] ); useEffect(() => { const fetchPaymentMethods = async () => { const url = "https://online-ordering.com/api/payment-methods"; const reaction = look forward to fetch(url); const tricks: RemotePaymentMethod[] = look forward to reaction.json(); if (tricks.period > 0) { const prolonged: LocalPaymentMethod[] = tricks.map((approach) => ({ supplier: approach.title, label: `Pay with ${approach.title}`, })); prolonged.push({ supplier: "money", label: "Pay in money" }); setPaymentMethods(prolonged); } else { setPaymentMethods([]); } }; fetchPaymentMethods(); }, []); go back { paymentMethods, }; };
Observe the nameless serve as inside of tricks.map
does the conversion
silently, and this common sense, at the side of the approach.supplier === "money"
above can also be extracted into a category.
We may have a category PaymentMethod
with the knowledge and behavior
centralised right into a unmarried position:
src/PaymentMethod.tsâ¦
magnificence PaymentMethod {
non-public remotePaymentMethod: RemotePaymentMethod;
constructor(remotePaymentMethod: RemotePaymentMethod) {
this.remotePaymentMethod = remotePaymentMethod;
}
get supplier() {
go back this.remotePaymentMethod.title;
}
get label() {
if(this.supplier === 'money') {
go back `Pay in ${this.supplier}`
}
go back `Pay with ${this.supplier}`;
}
get isDefaultMethod() {
go back this.supplier === "money";
}
}
With the category, I will be able to outline the default money fee approach:
const payInCash = new PaymentMethod({ title: "money" });
And right through the conversion – after the fee tricks are fetched from
the far off provider – I will be able to assemble the PaymentMethod
object in-place. And even
extract a small serve as known as convertPaymentMethods
:
src/usePaymentMethods.tsâ¦
const convertPaymentMethods = (tricks: RemotePaymentMethod[]) => {
if (tricks.period === 0) {
go back [];
}
const prolonged: PaymentMethod[] = tricks.map(
(approach) => new PaymentMethod(approach)
);
prolonged.push(payInCash);
go back prolonged;
};
Additionally, within the PaymentMethods
factor, we donât use the
approach.supplier === "money"
to test anymore, and as an alternative name the
getter
:
src/PaymentMethods.tsxâ¦
export const PaymentMethods = ({ choices }: { choices: PaymentMethod[] }) => (
<>
{choices.map((approach) => (
<label key={approach.supplier}>
<enter
kind="radio"
title="fee"
worth={approach.supplier}
defaultChecked={approach.isDefaultMethod}
/>
<span>{approach.label}</span>
</label>
))}
</>
);
Now weâre restructuring our Fee
factor into a number of smaller
portions that paintings in combination to complete the paintings.
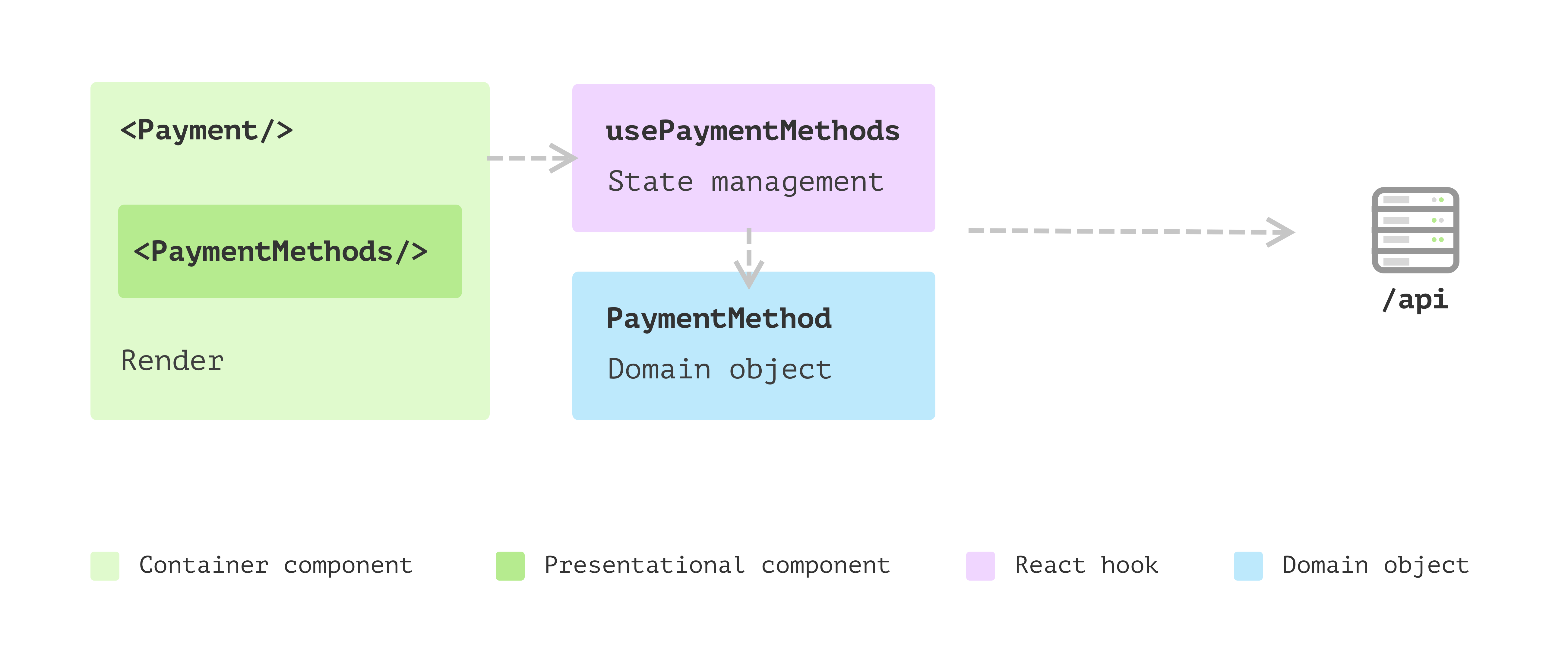
Determine 7: Refactored Fee with extra portions that may be composed simply
Some great benefits of the brand new construction
- Having a category encapsulates all of the common sense round a fee approach. Itâs a
area object and doesnât have any UI-related knowledge. So checking out and
doubtlessly enhancing common sense right here is far more uncomplicated than when embedded in a
view. - The brand new extracted factor
PaymentMethods
is a natural serve as and simplest
is dependent upon a site object array, which makes it tremendous simple to check and reuse
in other places. We may want to move in aonSelect
callback to it, however even in
that case, itâs a natural serve as and doesnât have to the touch any exterior
states. - Each and every a part of the function is obvious. If a brand new requirement comes, we will
navigate to the proper position with out studying all of the code.
I’ve to make the instance on this article sufficiently advanced in order that
many patterns can also be extracted. A majority of these patterns and ideas are
there to lend a hand simplify our code’s adjustments.
New requirement: donate to a charity
Letâs read about the speculation right here with some additional adjustments to the
utility. The brand new requirement is that we wish to be offering an possibility for
consumers to donate a small sum of money as a tip to a charity alongside
with their order.
As an example, if the order quantity is $19.80, we ask in the event that they would really like
to donate $0.20. And if a person is of the same opinion to donate it, weâll display the full
quantity at the button.
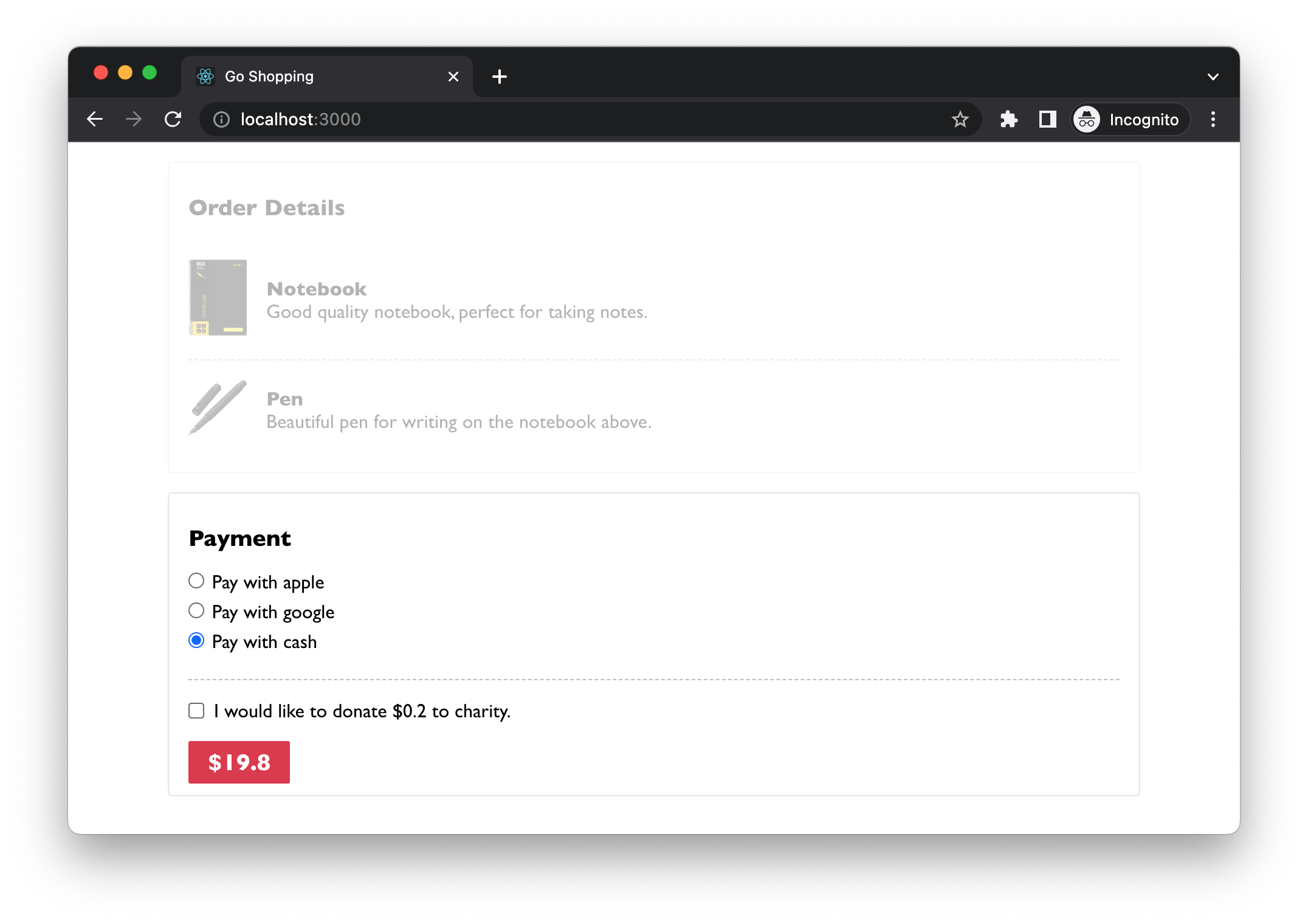
Determine 8: Donate to a charity
Prior to we make any adjustments, let’s have a handy guide a rough take a look at the present code
construction. I favor have other portions of their folder so it is simple for
me to navigate when it grows larger.
src âââ App.tsx âââ parts â âââ Fee.tsx â âââ PaymentMethods.tsx âââ hooks â âââ usePaymentMethods.ts âââ fashions â âââ PaymentMethod.ts âââ varieties.ts
App.tsx
is the primary access, it makes use of Fee
factor, and Fee
makes use of PaymentMethods
for rendering other fee choices. The hook
usePaymentMethods
is liable for fetching records from far off provider
after which convert it to a PaymentMethod
area object this is used to
dangle label
and the isDefaultChecked
flag.
Inner state: conform to donation
To make those adjustments in Fee
, we’d like a boolean state
agreeToDonate
to signify whether or not a person decided on the checkbox at the
web page.
src/Fee.tsxâ¦
const [agreeToDonate, setAgreeToDonate] = useState<boolean>(false); const { general, tip } = useMemo( () => ({ general: agreeToDonate ? Math.flooring(quantity + 1) : quantity, tip: parseFloat((Math.flooring(quantity + 1) - quantity).toPrecision(10)), }), [amount, agreeToDonate] );
The serve as Math.flooring
will around the quantity down so we will get the
right kind quantity when the person selects agreeToDonate
, and the variation
between the rounded-up worth and the unique quantity will probably be assigned to tip
.
And for the view, the JSX will probably be a checkbox plus a brief
description:
src/Fee.tsxâ¦
go back ( <div> <h3>Fee</h3> <PaymentMethods choices={paymentMethods} /> <div> <label> <enter kind="checkbox" onChange={handleChange} checked={agreeToDonate} /> <p> {agreeToDonate ? "Thank you in your donation." : `I want to donate $${tip} to charity.`} </p> </label> </div> <button>${general}</button> </div> );
With those new adjustments, our code begins dealing with more than one issues once more.
Itâs crucial to stick alert for doable blending of view and non-view
code. Should you to find any needless blending, search for tactics to separate them.
Observe that it isn’t a set-in-stone rule. Stay issues all in combination great
and tidy for small and cohesive parts, so that you would not have to appear in
more than one puts to grasp the entire behaviour. Typically, you must
remember to keep away from the factor document rising too giant to realize.
Extra adjustments about round-up common sense
The round-up appears just right thus far, and because the trade expands to different
nations, it comes with new necessities. The similar common sense doesnât paintings in
Japan marketplace as 0.1 Yen is simply too small as a donation, and it must around
as much as the closest hundred for the Jap forex. And for Denmark, it
must around as much as the closest tens.
It seems like a very simple repair. All I would like is a countryCode
handed into
the Fee
factor, proper?
<Fee quantity={3312} countryCode="JP" />;
And since all the common sense is now outlined within the useRoundUp
hook, I
too can move the countryCode
thru to the hook.
const useRoundUp = (quantity: quantity, countryCode: string) => { //... const { general, tip } = useMemo( () => ({ general: agreeToDonate ? countryCode === "JP" ? Math.flooring(quantity / 100 + 1) * 100 : Math.flooring(quantity + 1) : quantity, //... }), [amount, agreeToDonate, countryCode] ); //... };
You’re going to realize that the if-else can cross on and on as a brand new
countryCode
is added within the useEffect
block. And for the
getTipMessage
, we’d like the similar if-else tests as a special nation
might use different forex signal (as an alternative of a buck signal by way of default):
const formatCheckboxLabel = ( agreeToDonate: boolean, tip: quantity, countryCode: string ) => { const currencySign = countryCode === "JP" ? "Â¥" : "$"; go back agreeToDonate ? "Thank you in your donation." : `I want to donate ${currencySign}${tip} to charity.`; };
One final thing we additionally want to trade is the forex signal at the
button:
<button> {countryCode === "JP" ? "Â¥" : "$"} {general} </button>;
The shotgun surgical procedure downside
This situation is the well-known âshotgun surgical procedureâ scent we see in
many puts (no longer specifically in React packages). This necessarily
says that we will have to the touch a number of modules each time we want to regulate
the code for both a worm solving or including a brand new function. And certainly, itâs
more uncomplicated to make errors with this many adjustments, particularly when your checks
are inadequate.
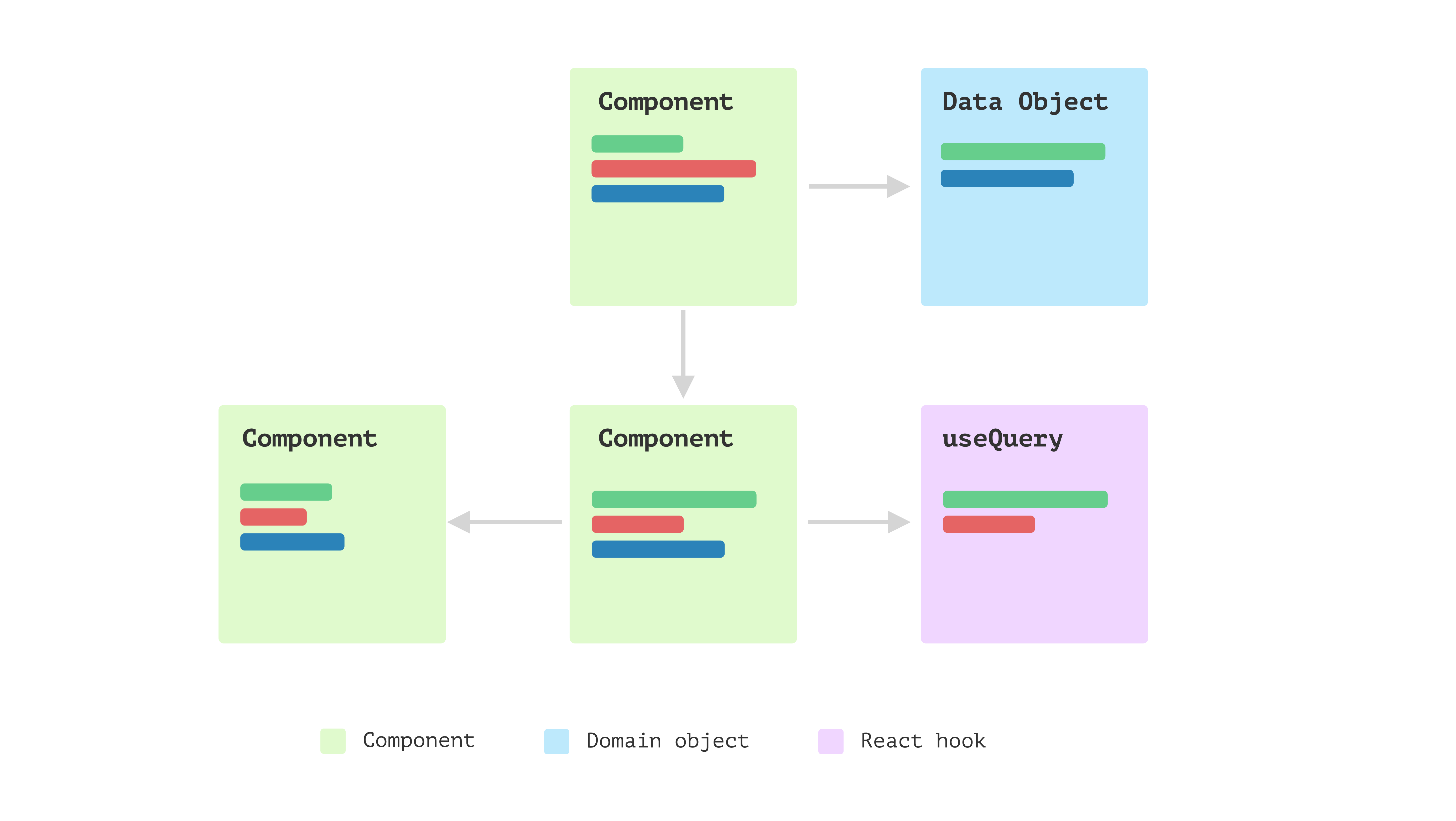
Determine 10: The shotgun surgical procedure scent
As illustrated above, the colored traces point out branches of nation
code tests that pass many recordsdata. In perspectives, weâll want to do separate
issues for various nation code, whilst in hooks, weâll want identical
branches. And each time we want to upload a brand new nation code, weâll need to
contact these kinds of portions.
As an example, if we believe Denmark as a brand new nation the trade is
increasing to, weâll finally end up with code in lots of puts like:
const currencySignMap = { JP: "Â¥", DK: "Kr.", AU: "$", }; const getCurrencySign = (countryCode: CountryCode) => currencySignMap[countryCode];
One imaginable resolution for the issue of getting branches scattered in
other puts is to make use of polymorphism to switch those transfer circumstances or
desk look-up common sense. We will be able to use Extract Magnificence on the ones
homes after which Substitute Conditional with Polymorphism.
Polymorphism to the rescue
The very first thing we will do is read about all of the permutations to look what
want to be extracted into a category. As an example, other nations have
other forex indicators, so getCurrencySign
can also be extracted right into a
public interface. Additionally ,nations may have other round-up
algorithms, thus getRoundUpAmount
and getTip
can cross to the
interface.
export interface PaymentStrategy { getRoundUpAmount(quantity: quantity): quantity; getTip(quantity: quantity): quantity; }
A concrete implementation of the tactic interface can be like
following the code snippet: PaymentStrategyAU
.
export magnificence PaymentStrategyAU implements PaymentStrategy {
get currencySign(): string {
go back "$";
}
getRoundUpAmount(quantity: quantity): quantity {
go back Math.flooring(quantity + 1);
}
getTip(quantity: quantity): quantity {
go back parseFloat((this.getRoundUpAmount(quantity) - quantity).toPrecision(10));
}
}
Observe right here the interface and categories don’t have anything to do with the UI
immediately. This common sense can also be shared elsewhere within the utility or
even moved to backend services and products (if the backend is written in Node, for
instance).
We may have subclasses for every nation, and every has the rustic explicit
round-up common sense. On the other hand, as serve as is top quality citizen in JavaScript, we
can move within the round-up set of rules into the tactic implementation to make the
code much less overhead with out subclasses. And becaues we have now just one
implementation of the interface, we will use Inline Magnificence to
cut back the single-implementation-interface.
src/fashions/CountryPayment.tsâ¦
export magnificence CountryPayment {
non-public readonly _currencySign: string;
non-public readonly set of rules: RoundUpStrategy;
public constructor(currencySign: string, roundUpAlgorithm: RoundUpStrategy) {
this._currencySign = currencySign;
this.set of rules = roundUpAlgorithm;
}
get currencySign(): string {
go back this._currencySign;
}
getRoundUpAmount(quantity: quantity): quantity {
go back this.set of rules(quantity);
}
getTip(quantity: quantity): quantity {
go back calculateTipFor(this.getRoundUpAmount.bind(this))(quantity);
}
}
As illustrated underneath, as an alternative of rely on scattered common sense in
parts and hooks, they now simplest depend on a unmarried magnificence
PaymentStrategy
. And at runtime, we will simply replace one example
of PaymentStrategy
for any other (the purple, inexperienced and blue sq. signifies
other cases of PaymentStrategy
magnificence).
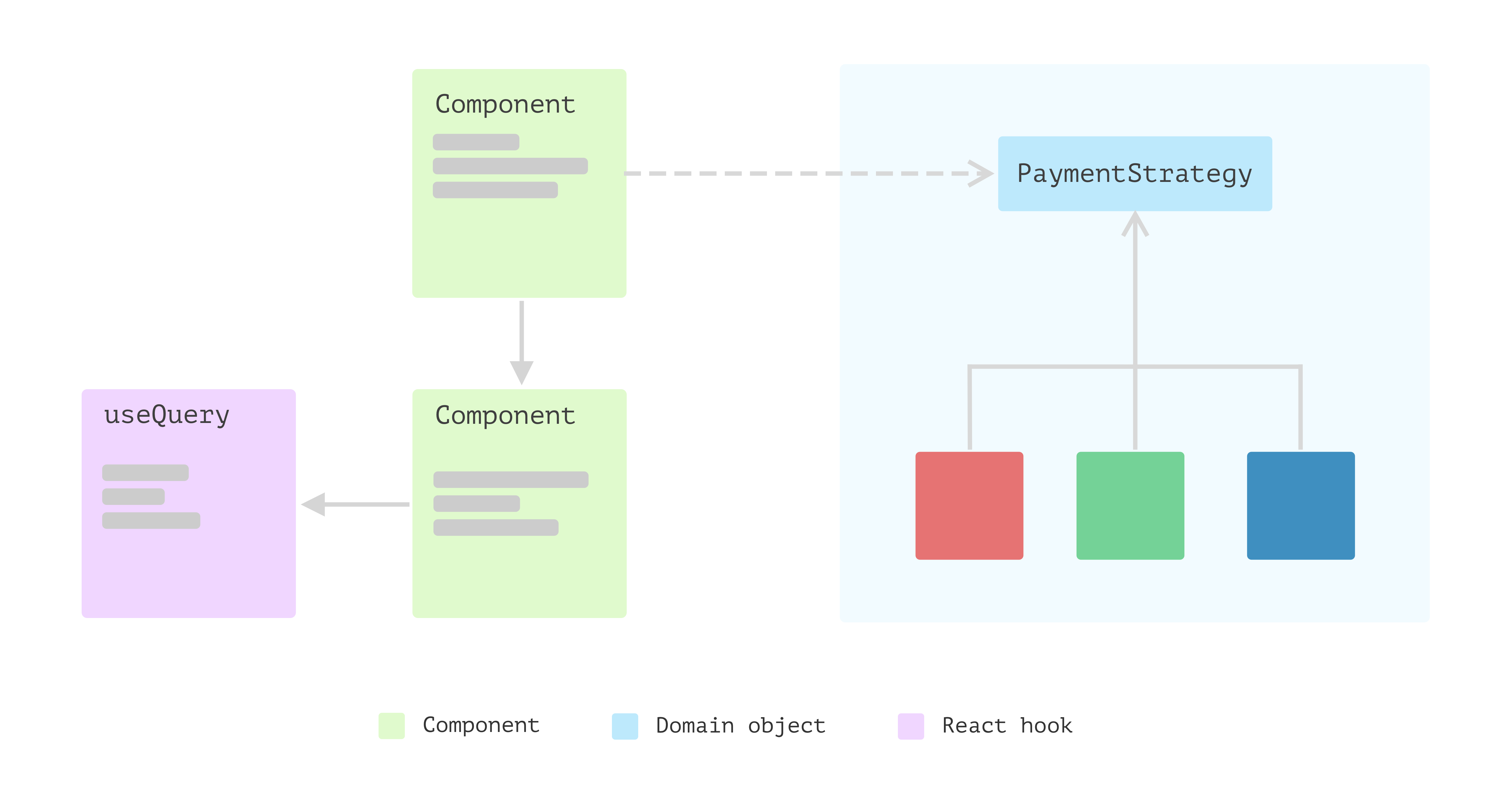
Determine 11: Extract magnificence to encapsulate common sense
And the useRoundUp
hook, the code might be simplified as:
src/hooks/useRoundUp.tsâ¦
export const useRoundUp = (quantity: quantity, technique: PaymentStrategy) => { const [agreeToDonate, setAgreeToDonate] = useState<boolean>(false); const { general, tip } = useMemo( () => ({ general: agreeToDonate ? technique.getRoundUpAmount(quantity) : quantity, tip: technique.getTip(quantity), }), [agreeToDonate, amount, strategy] ); const updateAgreeToDonate = () => { setAgreeToDonate((agreeToDonate) => !agreeToDonate); }; go back { general, tip, agreeToDonate, updateAgreeToDonate, }; };
Within the Fee
factor, we move the tactic from props
thru
to the hook:
src/parts/Fee.tsxâ¦
export const Fee = ({ quantity, technique = new PaymentStrategy("$", roundUpToNearestInteger), }: { quantity: quantity; technique?: PaymentStrategy; }) => { const { paymentMethods } = usePaymentMethods(); const { general, tip, agreeToDonate, updateAgreeToDonate } = useRoundUp( quantity, technique ); go back ( <div> <h3>Fee</h3> <PaymentMethods choices={paymentMethods} /> <DonationCheckbox onChange={updateAgreeToDonate} checked={agreeToDonate} content material={formatCheckboxLabel(agreeToDonate, tip, technique)} /> <button>{formatButtonLabel(technique, general)}</button> </div> ); };
And I then did just a little blank as much as extract a couple of helper purposes for
producing the labels:
src/utils.tsâ¦
export const formatCheckboxLabel = ( agreeToDonate: boolean, tip: quantity, technique: CountryPayment ) => { go back agreeToDonate ? "Thank you in your donation." : `I want to donate ${technique.currencySign}${tip} to charity.`; };
I am hoping you’ve spotted that weâre looking to immediately extract non-view
code into separate puts or summary new mechanisms to reform it to be
extra modular.
You’ll bring to mind it this manner: the React view is simplest one of the vital
shoppers of your non-view code. As an example, should you would construct a brand new
interface – perhaps with Vue or perhaps a command line software – how a lot code
are you able to reuse together with your present implementation?
Some great benefits of having those layers
As demonstrated above, those layers brings us many benefits:
- Enhanced maintainability: by way of setting apart an element into distinct portions,
it’s more uncomplicated to find and connect defects in explicit portions of the code. This may
save time and cut back the chance of introducing new insects whilst making adjustments. - Larger modularity: the layered construction is extra modular, which will
enable you to reuse code and construct new options. Even in every layer, take
perspectives for instance, have a tendency to be extra composable. - Enhanced clarity: it is a lot more uncomplicated to grasp and observe the common sense
of the code. This can also be particularly useful for different builders who’re studying
and dealing with the code. That is the core of constructing adjustments to the
codebase. - Stepped forward scalability: with diminished complixity in every person module,
the appliance is incessantly extra scalable, as it’s more uncomplicated so as to add new options or
make adjustments with out affecting all the device. This can also be particularly
necessary for massive, advanced packages which can be anticipated to adapt over
time. - Migrate to different techstack: if we need to (even most unlikely in maximum
tasks), we will change the view layer with out converting the underlying fashions
and common sense. All for the reason that area common sense is encapsulated in natural JavaScript (or
TypeScript) code and is not acutely aware of the lifestyles of perspectives.
Conclusion
Development React utility, or a frontend utility with React as its
view, must no longer be handled as a brand new form of tool. Lots of the patterns
and ideas for construction the normal person interface nonetheless follow. Even
the patterns for setting up a headless provider within the backend also are
legitimate within the frontend box. We will be able to use layers within the frontend and feature the
person interface as skinny as imaginable, sink the common sense right into a supporting mannequin
layer, and information get right of entry to into any other.
The good thing about having those layers in frontend packages is that you just
simplest want to perceive one piece with out being worried about others. Additionally, with
the advance of reusability, making adjustments to present code can be
rather extra manageable than ahead of.